create table BANKACCOUNT( id VARCHAR2(255) not null, name VARCHAR2(255) not null, balance NUMBER(10))tablespace TS_USER pctfree 10 initrans 1 maxtrans 255 storage ( initial 64K next 1M minextents 1 maxextents unlimited );alter table BANKACCOUNT add primary key (ID) using index tablespace TS_USER pctfree 10 initrans 2 maxtrans 255 storage ( initial 64K next 1M minextents 1 maxextents unlimited );2. 初始数据
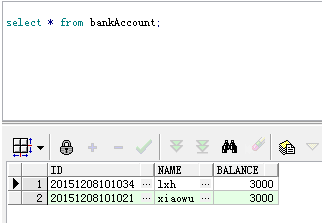
package com.lxh.transaction2;import java.sql.Connection;import java.sql.DriverManager;import java.sql.ResultSet;import java.sql.SQLException;import java.sql.Statement;import com.util.ConnectToDB;public class Test2 { public Connection conn = null; public Statement stat = null; public ResultSet rs = null; public static void main(String[] args) { Test2 t = new Test2(); try { /** * 数据库链接操作 */ Class.forName("oracle.jdbc.driver.OracleDriver"); t.conn = DriverManager.getConnection( "jdbc:oracle:thin:@127.0.0.1:1521:tran", "test", "123"); t.stat = t.conn.createStatement(); /********************** 转账 ***************************/ String sql1 = "update bankaccount set balance=3000-1000 where name='lxh'"; String sql2 = "update bankaccount set balance=3000+1000 where name='xiaowu'"; /** * 设置非自动提交----------非常重要 */ t.conn.setAutoCommit(false); // 批处理 t.stat.addBatch(sql1); t.stat.addBatch(sql2); /** * 处理执行SQL的结果 */ int res[] = t.stat.executeBatch(); int result = 1;// 正常执行,返回结果为1 for (int i : res) { result = i; } if (1 == result) { System.out.println("转账成功。。。"); // 执行结果全部为1的时候执行正确,允许提交 t.conn.commit(); t.conn.setAutoCommit(true); } else { // 不符合逻辑,回滚事务 System.out.println("转账失败。。。请核查输入参数"); t.conn.rollback(); } /********************** 转账 ***************************/ } catch (SQLException e) { System.out.println("转账失败:\t" + e.getMessage()); try { // 出现异常回滚(比如数据表名称/字段名称有误,参数有误) t.conn.rollback(); t.conn.setAutoCommit(true); } catch (SQLException e1) { e1.printStackTrace(); } } catch (ClassNotFoundException e) { System.out.println(e.getMessage()); } finally { // 关闭资源----其实就是将资源的关闭封装成类 ConnectToDB.closeResultSet(t.rs); ConnectToDB.closeStatemet(t.stat); ConnectToDB.closeConnection(t.conn); } }}4. 运行结果 <1> 账户名不存在 ( sql2= "update bankaccount set balance=3000+1000 where name='xiaowu1'")
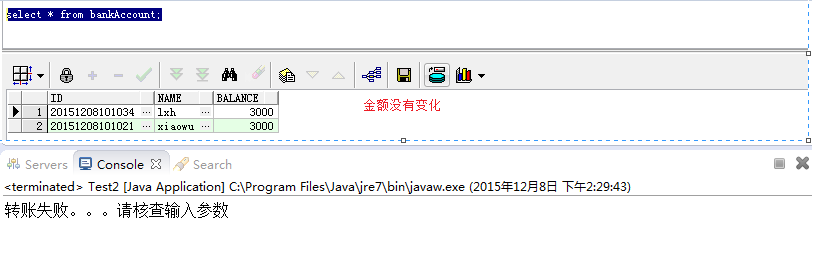
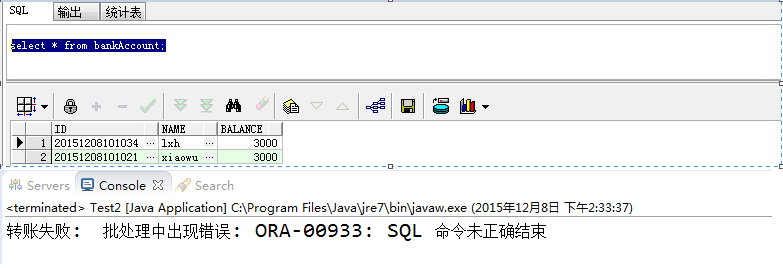
